Webflow Tutorial
How to Make an API Call in Webflow and Display the Results on the Page
.avif)
Join us in this practical tutorial where we demonstrate how to seamlessly integrate API calls into your Webflow projects and display the data directly on your web pages. Whether you're looking to fetch real-time data or enhance your site with dynamic content, this video covers all the steps necessary to make it happen. We'll walk you through the entire process, from setting up the API call to manipulating and displaying the data using custom code in Webflow. Perfect for web developers and enthusiasts looking to expand their Webflow capabilities with real-world applications.
All the code will be provided for you to follow along and implement in your own projects. Don't miss out on making your websites more interactive and informative with API data!
Disclaimer: This is a free API without a key. Don't use this method if you have a private API key.
API Call Code
Here is the code you will need in order to make the call to the api, pull the information into Webflow, display it on the page and scroll into view using GSAP.
document.addEventListener("DOMContentLoaded", function() {
// Register GSAP's ScrollTrigger plugin
gsap.registerPlugin(ScrollTrigger);
const quoteWrapper = document.querySelector(".quote_wrapper-api");
const baseURL = "https://api.quotable.io";
async function quote(endpoint, tag) {
try {
//check if a tag exists or not
const url = tag
? `${baseURL}/${endpoint}?tags=${tag}`
: `${baseURL}/${endpoint}`;
// fetch random quote from API
let response = await fetch(url);
// handle error if API is not reachable
if (!response.ok) throw new Error("Failed to fetch quote");
// destructure response - save author and content (quote)
const { author, content } = await response.json();
// return the author and content
return { author, content };
// catch block to catch any errors
} catch (error) {
console.error("Error fetching quote:", error);
return null;
}
}
// call async quote function. Parameters (endpoint, tag (filters quotes according to tag))
quote("random", "technology").then((quote) => {
console.log(quote.author, quote.content);
// checks to make sure a quote exists
if (quote) {
// find HTML elements
const quoteContent = document.querySelector(".quote_text-api"); //quote content
const quoteAuthor = document.querySelector(".quote_author-api"); //quote author
// Set text content of these elements
quoteContent.textContent = quote.content;
quoteAuthor.textContent = quote.author;
// if no quote
} else {
console.log("No quote found or there was an error fetching the quote");
}
});
gsap.to(quoteWrapper, {
opacity: 1,
duration: 1,
ease: "power1.in",
scrollTrigger: {
trigger: quoteWrapper,
start: "top 80%",
end: "bottom 70%",
toggleActions: "play none none none",
},
});
});
Breakdown of the Example Standard API Call
Certainly! The example you've provided is a comprehensive approach to making API calls, handling responses, and interacting with the UI based on the data retrieved. Below, I’ll break down how this script works and provide detailed explanations of each part, which you can refer to in your notes as a standard template for making API calls.
Detailed Breakdown of the API Call
Setting Up the Environment
- HTML Element Selection: const quoteWrapper = document.querySelector(".quote_wrapper-api");
- Purpose: Selects the HTML element with the class .quote_wrapper-api. This element acts as a container for displaying the quote content and author. It is also used as a target for animations via GSAP later in the script.
- Base URL Definition:const baseURL = "<https://api.quotable.io>";
- Purpose: Defines the base URL for the API calls. This is the root address of the API from which the data is fetched.
Defining the Asynchronous Function
- Function Definition:async function quote(endpoint, tag) {
...
}- Purpose: Declares an asynchronous function named quote that takes two parameters: endpoint and tag. The function is designed to fetch data from the API based on these parameters.
- Parameters:
- endpoint: The specific API endpoint to which the request is sent.
- tag: Optional parameter used to filter the quotes by a specific tag.
Constructing the URL and Fetching Data
- URL Construction:const url = tag ? `${baseURL}/${endpoint}?tags=${tag}` : `${baseURL}/${endpoint}`;
- Purpose: Constructs the URL for the fetch request. If a tag is provided, it appends a query parameter to filter the results by that tag.
- Fetch Request:let response = await fetch(url);
- Purpose: Makes the actual API call to the constructed URL using fetch. The await keyword pauses execution until the fetch completes, ensuring the next lines of code have the response data to work with.
Error Handling and Response Processing
- Error Handling:if (!response.ok) throw new Error("Failed to fetch quote");
- Purpose: Checks if the fetch was successful. If not, it throws an error which is caught by the catch block.
- Data Parsing and Destructuring:const { author, content } = await response.json();
- Purpose: Parses the JSON response from the API and destructures it to extract the author and content of the quote.
Returning and Using the Data
- Return Statement:return { author, content };
- Purpose: Returns an object containing the author and content, which can then be used elsewhere in the script or in other components.
- Using the Fetched Data:quote("random", "technology").then((quote) => {
...
});- Purpose: Calls the quote function with specific arguments and handles the returned promise. It logs the data and updates the UI if the quote exists.
UI Updates and Animations
- Updating HTML Elements:
const quoteContent = document.querySelector(".quote_text-api");
const quoteAuthor = document.querySelector(".quote_author-api");
quoteContent.textContent = quote.content;
quoteAuthor.textContent = quote.author;
- Purpose: Selects specific elements for displaying the quote and its author and sets their text content based on the data fetched.
- GSAP Animation:
gsap.to(quoteWrapper, {
...
});
- Purpose: Applies an animation to the quoteWrapper element using GSAP. The animation fades in the element based on its position in the viewport, enhanced by ScrollTrigger.
Summary
This script effectively demonstrates how to make API calls using asynchronous JavaScript, handle various outcomes (success and error scenarios), parse and use the data to update the UI, and apply animations for enhanced user interaction. It is a solid template for handling API interactions in web applications, providing a clear structure for fetching, processing, and displaying data dynamically.
HTML
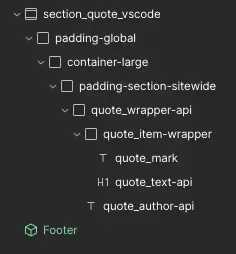
Head Section Code
Don't Forget to add this to the head section to set the quote wrapper to an opacity of O. As well as to pull in the GSAP Library and Scroll Trigger
<style>
.quote_wrapper-api {
opacity: 0; /* Start with invisible for transition */
}
</style>
<script defer src="https://cdnjs.cloudflare.com/ajax/libs/gsap/3.9.1/gsap.min.js"></script>
<script defer src="https://cdnjs.cloudflare.com/ajax/libs/gsap/3.9.1/ScrollTrigger.min.js"></script>
End to End Webflow Design and Development Services
From Web Design and SEO Optimization to Photography and Brand Strategy, we offer a range of services to cover all your digital marketing needs.
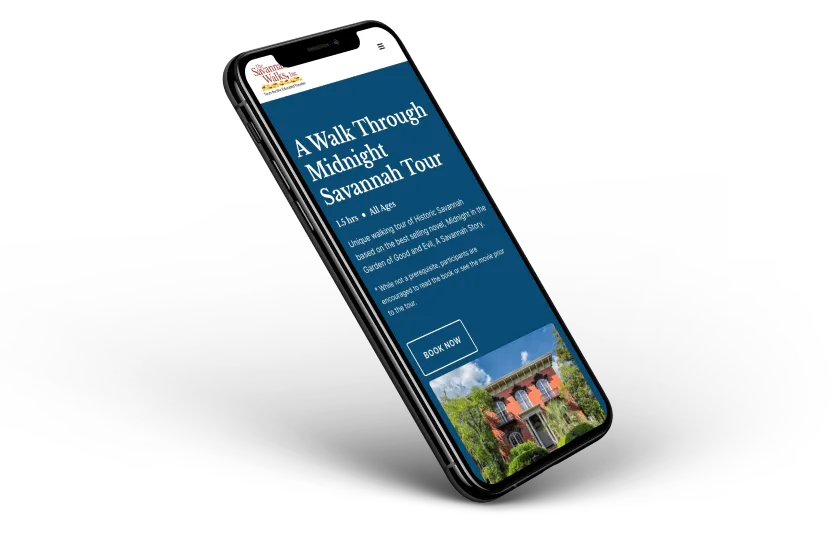
Webflow Web Design
We design custom Webflow websites that are unique, SEO optimized, and designed to convert.
Webflow Maintenance
Gain peace of mind knowing that a Webflow Professional Partner is maintaining your website.

Claim Your Design Spot Today
We dedicate our full attention and expertise to a select few projects each month, ensuring personalized service and results.
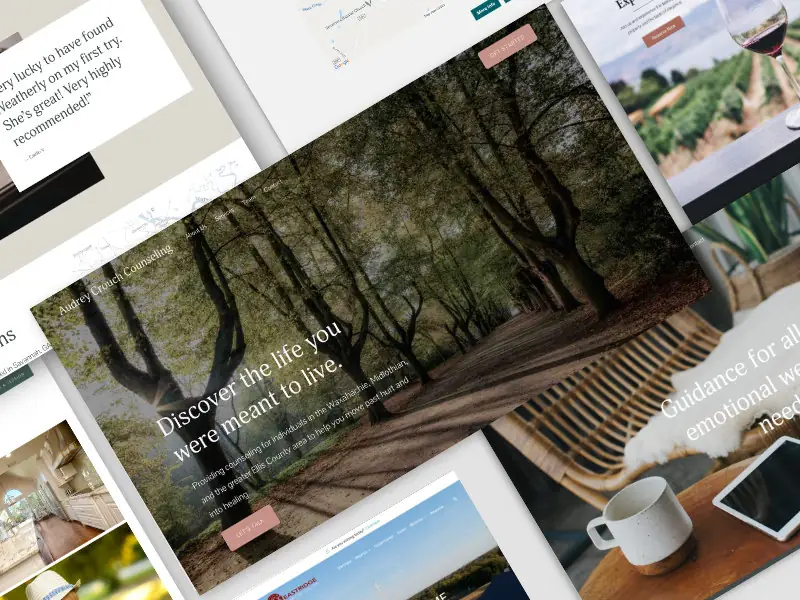