All Definitions
App Shell
Short Definition
A design strategy for Progressive Web Apps that separates static content from dynamic content for faster loading.
Definition
An App Shell is a web development architecture that provides a minimal HTML, CSS, and JavaScript structure required to power the user interface of a web application.
It ensures that the core UI elements load quickly and are cached for offline use, enabling a responsive and smooth user experience.
The App Shell model is commonly used in Progressive Web Apps (PWAs) to deliver a native app-like experience on the web.
When should you use an App Shell?
You should use an App Shell when:
- Developing Progressive Web Apps (PWAs) that require fast, reliable performance and offline capabilities.
- Creating single-page applications (SPAs) where a consistent and responsive user interface is crucial.
- Aiming to improve the loading performance of your web application by caching the essential UI elements.
- Providing a seamless user experience by ensuring the core interface loads quickly, even with slow network connections.
How should you use an App Shell?
To use an App Shell effectively, follow these steps:
- Identify Core UI Elements: Determine the essential components of your application's UI, such as navigation bars, headers, footers, and primary layout structures.
- Create the App Shell: Develop a minimal HTML, CSS, and JavaScript structure that includes the core UI elements. This structure should be lightweight and load quickly.
- Implement Caching: Use a service worker to cache the App Shell so that it can be served instantly on subsequent visits and available offline.
- Load Dynamic Content: Dynamically load and inject content into the App Shell as users interact with the application.
Example of a basic App Shell with a service worker:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>App Shell Example</title>
<link rel="stylesheet" href="styles.css">
<script defer src="app.js"></script>
</head>
<body>
<header>
<h1>My App</h1>
<nav>
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
</header>
<main id="content">
<!-- Dynamic content will be loaded here -->
</main>
<footer>
<p>© 2024 My App</p>
</footer>
<script>
// Register service worker
if ('serviceWorker' in navigator) {
navigator.serviceWorker.register('/service-worker.js').then(function(registration) {
console.log('Service Worker registered with scope:', registration.scope);
}).catch(function(error) {
console.log('Service Worker registration failed:', error);
});
}
</script>
</body>
</html>
Service Worker (service-worker.js):
const CACHE_NAME = 'app-shell-cache-v1';
const urlsToCache = [
'/',
'/styles.css',
'/app.js',
'/index.html'
];
self.addEventListener('install', function(event) {
event.waitUntil(
caches.open(CACHE_NAME)
.then(function(cache) {
return cache.addAll(urlsToCache);
})
);
});
self.addEventListener('fetch', function(event) {
event.respondWith(
caches.match(event.request)
.then(function(response) {
return response || fetch(event.request);
})
);
});
What is a real-world example of an App Shell in action?
A real-world example of an App Shell in action is Twitter's Progressive Web App (PWA). When users visit the Twitter PWA, the core UI elements, such as the navigation bar, header, and footer, load almost instantly.
This provides a consistent and responsive user experience, even before the dynamic content, such as tweets and user data, is loaded.
What are some precautions to take when working with an App Shell?
When working with an App Shell, consider the following precautions:
- Proper Caching: Ensure that the service worker correctly caches the App Shell and updates it when necessary to avoid serving outdated content.
- Network Requests: Optimize network requests to dynamically load content efficiently, avoiding excessive or redundant requests.
- User Feedback: Provide appropriate feedback, such as loading indicators, while dynamic content is being fetched and injected into the App Shell.
- Accessibility: Ensure that the App Shell and dynamically loaded content are accessible to all users, including those using assistive technologies.
What are the advantages of using an App Shell?
- Fast Load Times: Ensures that the core UI elements load quickly, improving the initial load time and user experience.
- Offline Support: Provides offline capabilities by caching the essential UI elements, allowing users to access the app without an internet connection.
- Consistent UI: Delivers a consistent and responsive user interface, similar to native apps.
- Improved Performance: Reduces the amount of data that needs to be loaded over the network, enhancing performance on slow connections.
What are the limitations of using an App Shell?
- Initial Complexity: Implementing an App Shell with service workers and caching can be complex and require additional setup.
- Content Updates: Ensuring that cached content is updated correctly can be challenging, especially if the App Shell changes frequently.
- SEO Challenges: Dynamically loaded content may not be indexed by search engines, potentially impacting SEO unless proper measures are taken.
What are common mistakes to avoid with an App Shell?
- Overloading the Shell: Including too much content in the App Shell can negate its performance benefits. Keep it minimal.
- Ignoring Updates: Failing to update the App Shell cache can lead to outdated or inconsistent UI elements.
- Poor Offline Experience: Not handling offline scenarios properly can result in broken links or missing content.
- Neglecting Accessibility: Overlooking accessibility can make the App Shell unusable for users with disabilities.
How does App Shell compare to similar technologies or methods?
- App Shell vs. Single-Page Application (SPA): Both aim to provide a smooth, responsive user experience. An App Shell is a strategy used within SPAs to ensure fast initial load times and offline capabilities.
- App Shell vs. Server-Side Rendering (SSR): SSR improves initial load times by rendering content on the server, while an App Shell focuses on caching the core UI elements on the client side. Both can be used together for optimal performance.
- App Shell vs. Traditional Web App: Traditional web apps load all content with each request, whereas an App Shell caches the core UI for faster subsequent loads and offline access.
What are best practices for App Shell?
- Keep it Minimal: Include only the essential UI elements in the App Shell to ensure quick load times.
- Implement Proper Caching: Use service workers to cache the App Shell and update it as needed.
- Optimize Dynamic Loading: Efficiently load and inject dynamic content into the App Shell to maintain performance.
- Ensure Accessibility: Make sure the App Shell and content are accessible to all users.
- Test Extensively: Regularly test the App Shell on different devices and network conditions to ensure a consistent user experience.
What resources are available for learning more about App Shell?
- MDN Web Docs: Comprehensive documentation on service workers, caching, and web app development.
- Google Developers: Guides and best practices for building Progressive Web Apps (PWAs) with an App Shell model.
- "Progressive Web Apps" by Jason Grigsby: A book covering the principles and implementation of PWAs, including the App Shell architecture.
- CSS-Tricks: Articles and tutorials on implementing App Shell and other performance optimization techniques.
- Web.dev: A resource for learning about modern web development practices, including PWAs and App Shell architecture.
By understanding and applying these aspects of the App Shell architecture, you can create fast, reliable, and user-friendly web applications that provide a seamless experience similar to native apps.
Keep Learning
Aspect Ratio
The ratio of the width to the height of an element, often used in image and video dimensions.
Content Delivery Network (CDN)
A system of distributed servers that deliver web content to a user based on their geographic location.
Ready to take your business to the next level?
Expand your reach and grow your business with our seamless integration of web design and expert SEO strategies. Apply now to secure your spot.
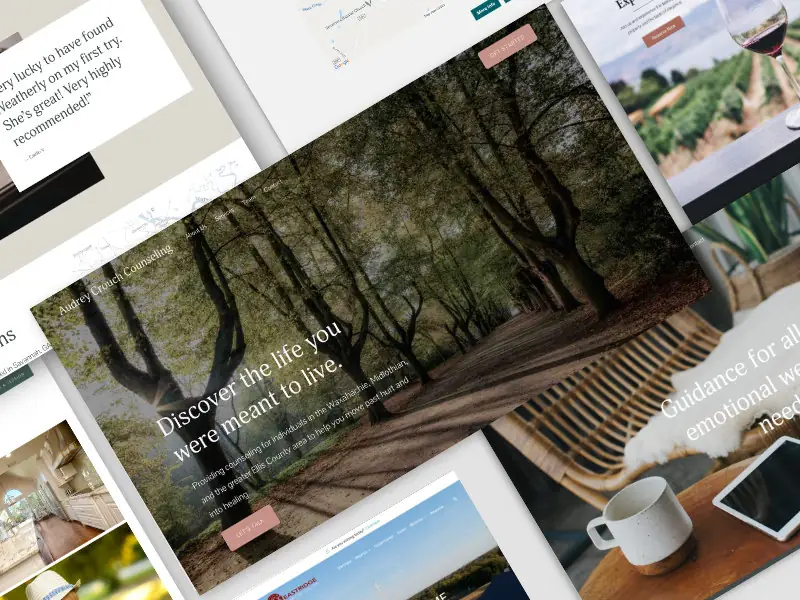