All Definitions
Contextual Menu
Short Definition
A menu that appears upon user interaction, offering relevant options based on the context of the action performed.
Definition
A Contextual Menu is a user interface element that provides a list of actions or options relevant to the specific context of the item or area the user is interacting with. It typically appears when the user right-clicks on an object or holds a tap on a touchscreen device. The options available in a contextual menu depend on what the user is interacting with, making it context-sensitive and more efficient for performing certain tasks.
When should you use a Contextual Menu?
You should use a Contextual Menu when you want to provide users with quick access to specific actions that are relevant to the object or area they are interacting with.
It is particularly useful in applications, websites, or software where users need to perform frequent actions, such as copying, pasting, renaming files, or accessing additional features.
Contextual menus are common in desktop applications, file management systems, and graphic design software, and can improve user productivity by offering on-the-spot actions.
How should you use a Contextual Menu?
To use a Contextual Menu effectively, follow these steps:
- Determine Context-Sensitive Actions: Identify the most relevant and frequently used actions for each object or section where the contextual menu will be applied.
- Trigger the Menu: Implement triggers for the menu, such as right-clicking on desktop interfaces or long presses on touchscreens.
- Organize the Menu: Group related actions together logically, such as editing options, navigation, or file actions.
- Keep it Simple: Limit the number of actions in the contextual menu to avoid overwhelming users, ensuring only context-relevant actions are included.
- Ensure Accessibility: Make sure the contextual menu is accessible via keyboard shortcuts or assistive technologies for users who may not use a mouse or touchscreen.
Example of a basic contextual menu in HTML using JavaScript:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Contextual Menu Example</title>
<style>
#context-menu {
display: none;
position: absolute;
background-color: white;
border: 1px solid #ccc;
padding: 10px;
}
#context-menu li {
list-style: none;
padding: 5px;
cursor: pointer;
}
</style>
</head>
<body>
<div id="container" style="width: 100%; height: 200px; border: 1px solid #000;">
Right-click inside this box for the contextual menu.
</div>
<ul id="context-menu">
<li>Copy</li>
<li>Paste</li>
<li>Delete</li>
</ul>
<script>
const container = document.getElementById('container');
const menu = document.getElementById('context-menu');
container.addEventListener('contextmenu', (e) => {
e.preventDefault();
menu.style.display = 'block';
menu.style.left = `${e.pageX}px`;
menu.style.top = `${e.pageY}px`;
});
window.addEventListener('click', () => {
menu.style.display = 'none';
});
</script>
</body>
</html>
What is a real-world example of a Contextual Menu in action?
A real-world example of a Contextual Menu is in desktop operating systems like Windows or macOS.
When you right-click on a file, a contextual menu appears with options such as "Open," "Copy," "Rename," "Delete," and "Properties."
These options are directly related to the selected file, making it easier for users to perform tasks without navigating through multiple menus.
What are some precautions to take when working with a Contextual Menu?
When working with a Contextual Menu, consider the following precautions:
- Avoid Overloading with Options: Too many actions in a contextual menu can overwhelm users. Keep the menu concise and focused on the context.
- Ensure Intuitive Use: Make sure users know the contextual menu is available, especially for touch-based devices where long-press actions may not be as obvious as right-clicking.
- Check for Accessibility: Ensure that users who rely on keyboard navigation or assistive technologies can access the contextual menu.
- Prevent Accidental Actions: Make sure critical actions (like "Delete" or "Move") require confirmation to avoid accidental actions.
- Cross-Browser Testing: Test the contextual menu across different browsers and devices to ensure consistent behavior and functionality.
What are the advantages of using a Contextual Menu?
- Context-Relevant Options: Provides users with options that are specifically related to the object or task they are interacting with, improving usability.
- Increased Efficiency: Allows users to perform actions quickly without navigating through multiple menus or toolbars.
- Cleaner UI: Reduces clutter in the interface by hiding secondary actions until needed, maintaining a streamlined and minimalist design.
- Intuitive Design: Users are accustomed to right-clicking or long-pressing for additional options, making contextual menus a familiar feature in modern interfaces.
- Task-Specific Customization: Allows customization of options based on the type of object selected, ensuring actions are highly relevant to the user.
What are the limitations of using a Contextual Menu?
- Discoverability: Not all users may know to right-click or long-press to access the contextual menu, especially on touch devices.
- Inaccessibility: If not designed properly, contextual menus may not be easily accessible to users with disabilities, particularly those who rely on keyboard navigation.
- Action Overload: Including too many options in the menu can lead to decision fatigue, causing users to overlook important actions.
- Limited Space: On smaller screens, such as mobile devices, contextual menus may have limited space to display options, affecting usability.
What are common mistakes to avoid with a Contextual Menu?
- Overloading with Unnecessary Options: Avoid including irrelevant or rarely used actions, which can overwhelm users and reduce the menu's effectiveness.
- Poorly Organized Actions: Ensure actions in the menu are grouped logically to help users quickly find what they need.
- Lack of Accessibility: Failing to make the menu accessible via keyboard shortcuts or assistive technologies can exclude certain users.
- No Feedback or Confirmation: Make sure critical actions, like "Delete," prompt the user for confirmation to avoid accidental actions.
- Inconsistent Design: Ensure that contextual menus have a consistent design and behavior across different parts of the application or website.
How does a Contextual Menu compare to similar technologies or methods?
- Contextual Menu vs. Dropdown Menu: A dropdown menu is usually tied to a specific button or navigation item, while a contextual menu appears in response to a right-click or long-press and offers context-sensitive options.
- Contextual Menu vs. Toolbar: Toolbars are always visible and provide a set of general actions, whereas a contextual menu appears only when necessary and offers actions relevant to the current context.
- Contextual Menu vs. Modal Window: A modal window requires user interaction to proceed, while a contextual menu provides quick options without disrupting the user's workflow.
- Contextual Menu vs. Hamburger Menu: A hamburger menu provides a list of navigation options, typically for an entire site or app, whereas a contextual menu focuses on a specific item or task.
What are best practices for Contextual Menus?
- Keep it Simple: Only include actions that are relevant to the context, keeping the menu clean and easy to navigate.
- Group Related Actions: Organize similar actions together to help users quickly find what they need.
- Ensure Keyboard Accessibility: Provide keyboard shortcuts or focusable elements so that users can access the menu without a mouse or touchscreen.
- Test on Different Devices: Make sure the contextual menu works well across all devices, including desktops, tablets, and smartphones.
- Provide Feedback for Critical Actions: For destructive actions like "Delete" or "Remove," ensure users receive a confirmation prompt to prevent accidental actions.
What resources are available for learning more about Contextual Menus?
- MDN Web Docs: Comprehensive documentation on creating and managing contextual menus in web applications.
- CSS-Tricks: Articles and tutorials on implementing contextual menus using HTML, CSS, and JavaScript.
- W3Schools: Examples and guides on creating right-click menus and other interactive elements.
- Smashing Magazine: Resources and best practices for creating intuitive and accessible contextual menus.
- "Don't Make Me Think" by Steve Krug: A book on usability that includes insights into creating efficient and user-friendly UI components, including contextual menus.
By understanding and applying these aspects of a Contextual Menu, you can enhance user experience by offering quick, relevant actions that streamline workflows, improve usability, and maintain a clean interface design.
Ready to take your business to the next level?
Expand your reach and grow your business with our seamless integration of web design and expert SEO strategies. Apply now to secure your spot.
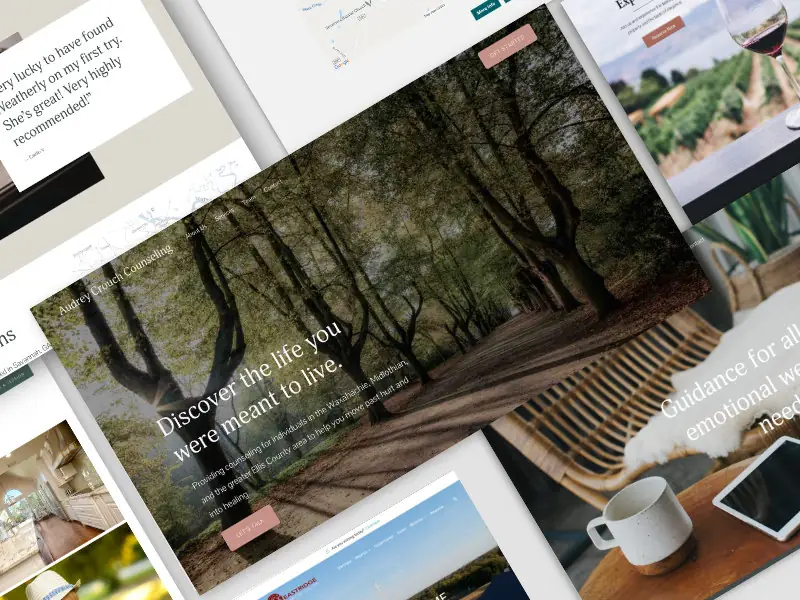